on:
push:
branches:
- main
pull_request:
jobs:
test:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v4
with:
fetch-depth: 0
- name: Secret Scanning
uses: trufflesecurity/trufflehog@main
with:
extra_args: --only-verified
In the example above, we're using it to check for any exposed secrets whenever someone makes a pull request or updates the main branch. It only looks at the code changes in those specific update.
TruffleHog
Find leaked credentials.
What is TruffleHog?
TruffleHog checks our code for any hidden keys or passwords that we might not realize are exposed. It goes through our old code to find anything sensitive that shouldn't be there.
We can use TruffleHog while we develop our software to make sure our project stays secure. It can automatically check every update we make to ensure we aren’t accidentally leaking any secrets. This could help us catch any security issues before our code is even officially released.
Shallow Cloning for Faster Checks
If you're setting up TruffleHog by itself and not using other CI/CD tools with it, we'd suggest using Shallow Cloning to make things run faster. Here's how you can do it:
We set it up so that, depending on whether it's a push or a pull request, it figures out how many commits are involved. Then, we add two to that number. This helps us look back to a commit just before the changes started. We use this number for the fetch-depth
in the checkout step, which makes the checkout process quicker because it doesn't download the entire history.
Here’s the setup:
- shell: bash
run: |
if [ "${{ github.event_name }}" == "push" ]; then
echo "depth=$(($(jq length <<< '${{ toJson(github.event.commits) }}') + 2))" >> $GITHUB_ENV
echo "branch=${{ github.ref_name }}" >> $GITHUB_ENV
fi
if [ "${{ github.event_name }}" == "pull_request" ]; then
echo "depth=$((${{ github.event.pull_request.commits }}+2))" >> $GITHUB_ENV
echo "branch=${{ github.event.pull_request.head.ref }}" >> $GITHUB_ENV
fi
- uses: actions/checkout@v3
with:
ref: ${{env.branch}}
fetch-depth: ${{env.depth}}
- uses: trufflesecurity/trufflehog@main
with:
extra_args: --only-verified
This setup should speed up the checking process by focusing only on the recent changes rather than the entire project history.
Canary Detection for Safer Scanning
Canary detection is a security measure that uses decoy tokens, called canaries, to alert you if someone accesses your systems or data in an unauthorized way. When these canaries are triggered, they send an alert, letting you know there might be a security breach without the intruder knowing they've been detected.
We can use Canary detection with TruffleHog. It's pretty cool because it can spot what are called canary tokens in our code. The best part is, it detects these tokens without activating them, so we don't have to worry about setting off any alarms while checking.
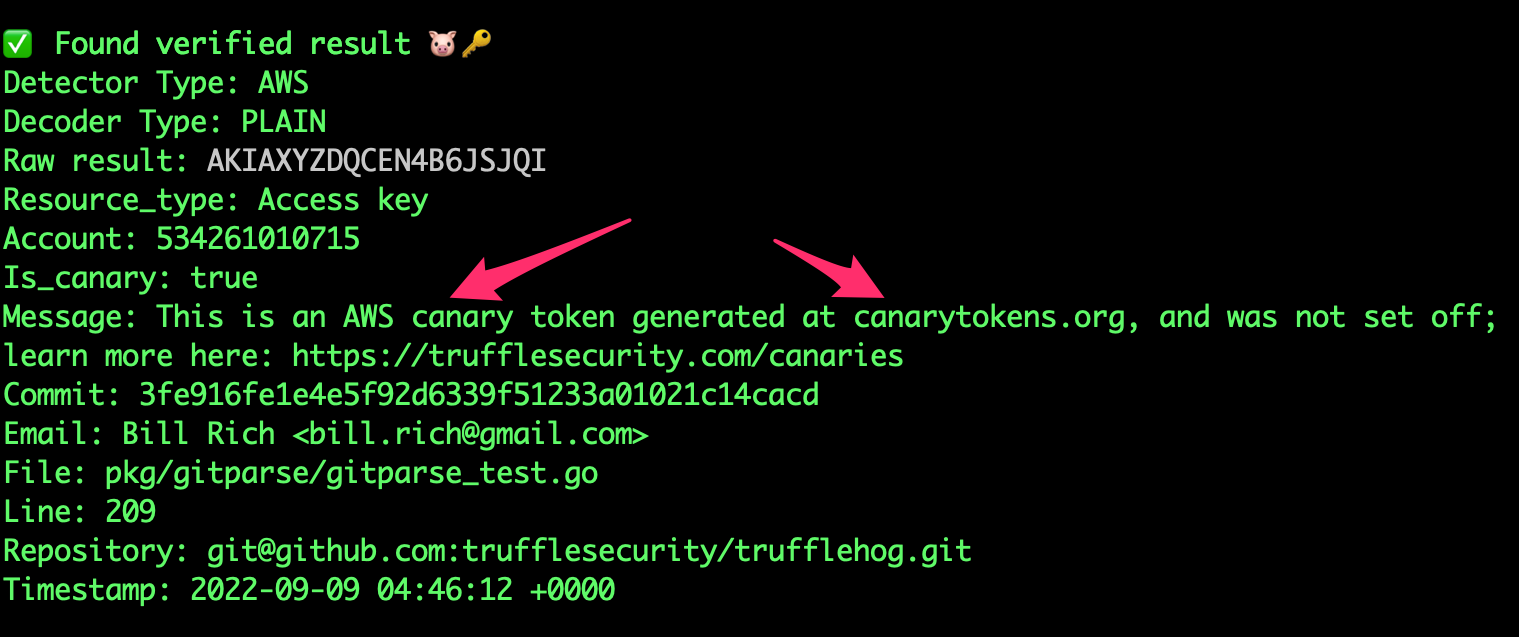
If you're curious and want to dive deeper into how it works and what benefits it offers, you can check out more details here: https://trufflesecurity.com/canaries
Advanced Usage for Specific Cases
If you're looking to take our TruffleHog implementation to the next level with some advanced settings, here's how you can set it up in our GitHub Actions for more specific cases:
- name: TruffleHog
uses: trufflesecurity/trufflehog@main
with:
# Specify the repository path if not default
path:
# Start scanning from this branch (commonly the main branch)
base:
# Scan up to this branch (commonly the development branch)
head: # optional
# Additional arguments for the TruffleHog command line
extra_args: --debug --only-verified
In this setup, you can define base
and head
to specify where you want to start and stop scanning within your repository history. For example, if you want to scan between specific commits or branches, you can use base
as your starting point (--since-commit
in CLI) and head
as your endpoint (--branch
in CLI).
This is useful for targeted scans, especially in larger repos or where changes are frequent.
Scan Entire Branch Configuration
If your goal is to scan the entire branch every time there's a push, here’s how you could configure it:
- name: scan-push
uses: trufflesecurity/trufflehog@main
with:
base: ""
head: ${{ github.ref_name }}
extra_args: --only-verified
This configuration initiates a scan from the base of the branch to the current reference point, which is particularly handy for ongoing security checks in active development branches.